프로젝트 구성
- IDE : Intellj
- JDK 1.8
- Maven Project (groupId = hello, artifactId = servlet, packaging = jar)
- Spring Boot 2.4.x
- Dependency (Spring Web, Lombok)
HelloServlet
전통적인 스프링 웹 애플리케이션은 web.xml에 서블릿을 등록했다. 하지만 톰캣 7(서블릿 3.0)부터 자바 애노테이션으로 서블릿을 등록할 수 있다. (톰캣이 @WebServlet이 적용된 클래스를 검색해 서블릿으로 등록한다.)
1. @ServletComponentScan 추가
프로젝트의 ServletApplicaion 클래스에 @ServletComponentScan 애노테이션을 추가한다. SpringBoot에서 제공하는 애노테이션으로 선언된 클래스가 포함된 패키지부터 그 하위 패키지까지 작성된 서블릿을 등록하고 사용할 수 있도록 한다.
package hello.servlet;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletComponentScan;
@ServletComponentScan //servlet auto register
@SpringBootApplication
public class ServletApplication {
public static void main(String[] args) {
SpringApplication.run(ServletApplication.class, args);
}
}
2. HelloServlet 생성
package hello.servlet.basic;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@WebServlet(name = "helloServlet", urlPatterns = "/hello")
public class HelloServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("name");
response.setContentType("text/plain");
response.setCharacterEncoding("utf-8");
response.getWriter().write("hello" + username);
}
}
결과 확인
http://127.0.0.1:8080/hello?name=jun URL로 요청을 보내면 아래의 응답 메세지를 확인할 수 있다.
HttpServletRequest
톰캣은 HTTP 요청 메세지를 파싱해 HttpServletRequest 객체에 담고 서블릿 service 메서드의 인자로 전달한다. HttpServletRequest 객체는 아래의 정보를 담고 있다.
- REQUEST LINE (HTTP 메서드, URL, Query String, Scheme, Protocol)
- Header
- Body (form 파라미터 형식 조회, message body 데이터 직접 조회)
HttpServletRequest와 HttpServletResponse 객체를 잘 활용하려면 HTTP 스펙을 알아두는 것이 중요하다.
HttpServletResponse
서블릿의 service 메서드에서 요청에 대한 응답 정보를 HttpServletResponse 객체에 담는다. 서블릿의 service 메서드가 끝나면 톰캣이 HttpServletResponse 객체로부터 응답 메세지를 만들어 응답한다.
HttpServletResponse 객체에 아래의 정보를 담는다.
- STATUS LINE
- Header
- Body
HTTP 요청 데이터
HTTP 요청을 보낼 때 데이터를 포함시킬 수 있는데 주로 3가지 방법을 사용한다.
GET - Query Parameter
- 요청 메세지 바디 없이 URL 쿼리 파라미터에 데이터를 포함해서 보내는 요청하는 방식
- 검색, 필터, 페이징 등에서 많이 사용된다.
POST - HTML Form
- HTML Form을 통해 데이터를 담아 요청하는 방식
- content-type 헤더 : application/x-www-form-urlencoded
- 요청 메세지 바디에 데이터를 쿼리 파라미터 형식으로 전달한다.
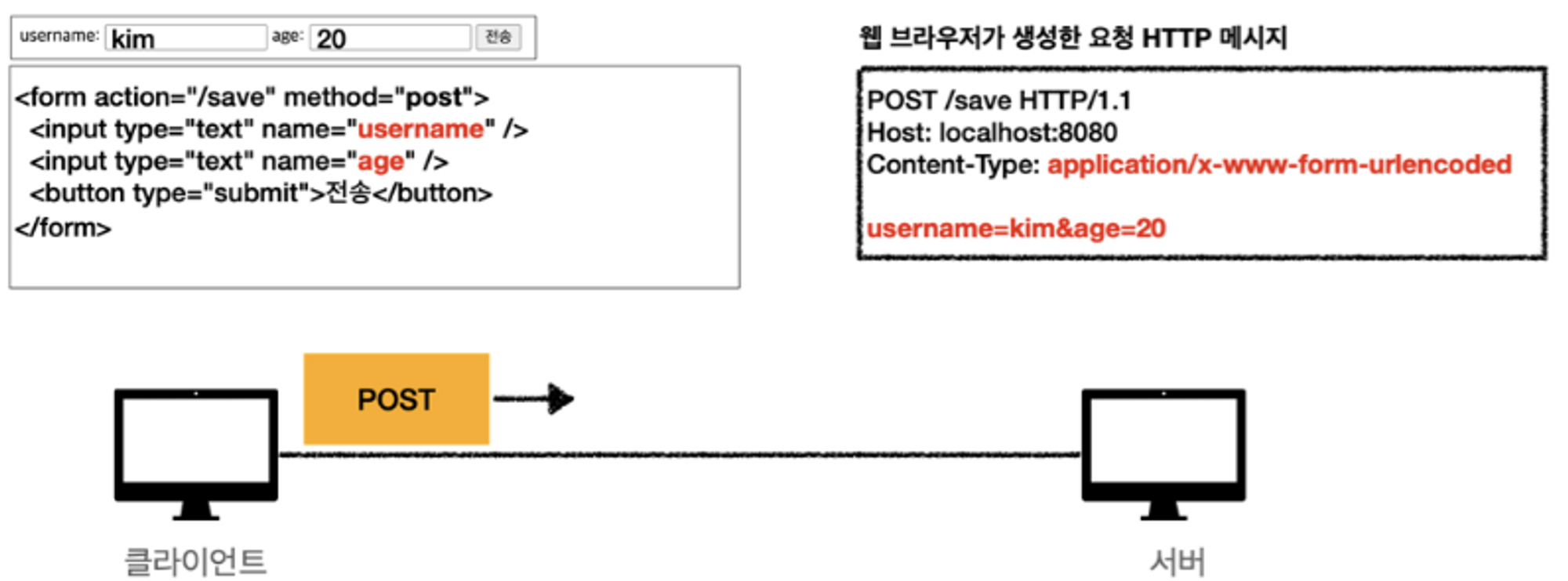
HTTP message body에 데이터를 담아 요청
- 요청 메세지 바디에 개발자가 직접 데이터를 담아 요청하는 방식
- 데이터 포맷으로 JSON, XML, TEXT를 주로 사용한다.
- HTTP 메서드로 POST, PUT, PATCH를 주로 사용한다.
HTTP 응답 데이터
응답 메세지의 바디에 응답 데이터가 담기는데 보통 JSON, 텍스트, HTML를 담는다.
'Spring > Spring MVC' 카테고리의 다른 글
스프링 검증 (1) (0) | 2023.03.27 |
---|---|
스프링 MVC 기본 기능 (0) | 2023.02.28 |
스프링 MVC (2) (0) | 2023.02.21 |
스프링 MVC (1) (0) | 2023.02.17 |
서블릿 (0) | 2023.02.14 |