Regex (정규식)
전체 문자열 또는 문자열의 substring이 어떤 패턴 또는 규칙을 만족하는지 알고싶을때 Regex (정규식)을 사용한다. Regex는 문자열의 패턴을 나타내는 언어이다.
Regex 기호
아래의 사이트에 잘 정리되어 있다. 왼쪽 메뉴의 CheatsSheet를 보고 실습하면서 기호를 파악하고 자세한 내용은 RegEx Reference를 참고하자.
RegExr: Learn, Build, & Test RegEx
RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp).
regexr.com
JAVA에서 사용
String 객체의 matches 메서드는 모든 문자열의 문자가 정규식에 매치되면 'true'를 아니면 'false'를 반환한다.
public class Main {
public static void main(String[] args) throws IOException {
String str1 = "AAAOA";
String str2 = "BBBBB";
String regex1 = "A+OA";
String regex2 = "^[A-Za-z]*$";
String regex3 = "((A|a)|(O|o))*";
System.out.println("REGEX1 RESULT");
if(str1.matches(regex1)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex1)) System.out.println("yes");
else System.out.println("no");
System.out.println();
System.out.println("REGEX2 RESULT");
if(str1.matches(regex2)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex2)) System.out.println("yes");
else System.out.println("no");
System.out.println();
System.out.println("REGEX3 RESULT");
if(str1.matches(regex3)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex3)) System.out.println("yes");
else System.out.println("no");
System.out.println();
}
}
문자열에서 정규식에 매칭되는 서브 문자열을 찾고 싶다면 Matcher 클래스를 사용하면 된다. 아래 코드는 숫자로만 구성된 서브 문자열의 시작 인덱스, 끝 인덱스, 서브 문자열을 출력한다.
public class Main {
public static void main(String[] args) throws IOException {
String str = "A1333CC444AAB55C66CC";
String regex = "[0-9]+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(str);
while(matcher.find()) {
int start = matcher.start();
int end = matcher.end();
System.out.println("start_idx : " + start + " " + "end_idx : " + end);
System.out.println(matcher.group());
}
str = str.replaceAll("[0-9]+", "X");
System.out.println(str);
}
}
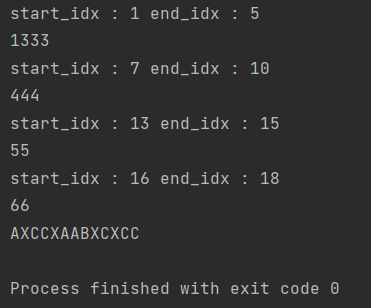
'Java > Basic' 카테고리의 다른 글
Java 람다식 (0) | 2023.04.05 |
---|---|
Java 익명 클래스 (0) | 2023.04.04 |
[JVM - 2] ClassLoader (0) | 2022.10.25 |
Java 정렬 (0) | 2022.09.07 |
[JVM - 1] JVM, JRE, JDK (0) | 2022.08.17 |
Regex (정규식)
전체 문자열 또는 문자열의 substring이 어떤 패턴 또는 규칙을 만족하는지 알고싶을때 Regex (정규식)을 사용한다. Regex는 문자열의 패턴을 나타내는 언어이다.
Regex 기호
아래의 사이트에 잘 정리되어 있다. 왼쪽 메뉴의 CheatsSheet를 보고 실습하면서 기호를 파악하고 자세한 내용은 RegEx Reference를 참고하자.
RegExr: Learn, Build, & Test RegEx
RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp).
regexr.com
JAVA에서 사용
String 객체의 matches 메서드는 모든 문자열의 문자가 정규식에 매치되면 'true'를 아니면 'false'를 반환한다.
public class Main {
public static void main(String[] args) throws IOException {
String str1 = "AAAOA";
String str2 = "BBBBB";
String regex1 = "A+OA";
String regex2 = "^[A-Za-z]*$";
String regex3 = "((A|a)|(O|o))*";
System.out.println("REGEX1 RESULT");
if(str1.matches(regex1)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex1)) System.out.println("yes");
else System.out.println("no");
System.out.println();
System.out.println("REGEX2 RESULT");
if(str1.matches(regex2)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex2)) System.out.println("yes");
else System.out.println("no");
System.out.println();
System.out.println("REGEX3 RESULT");
if(str1.matches(regex3)) System.out.println("yes");
else System.out.println("no");
if(str2.matches(regex3)) System.out.println("yes");
else System.out.println("no");
System.out.println();
}
}
문자열에서 정규식에 매칭되는 서브 문자열을 찾고 싶다면 Matcher 클래스를 사용하면 된다. 아래 코드는 숫자로만 구성된 서브 문자열의 시작 인덱스, 끝 인덱스, 서브 문자열을 출력한다.
public class Main {
public static void main(String[] args) throws IOException {
String str = "A1333CC444AAB55C66CC";
String regex = "[0-9]+";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(str);
while(matcher.find()) {
int start = matcher.start();
int end = matcher.end();
System.out.println("start_idx : " + start + " " + "end_idx : " + end);
System.out.println(matcher.group());
}
str = str.replaceAll("[0-9]+", "X");
System.out.println(str);
}
}
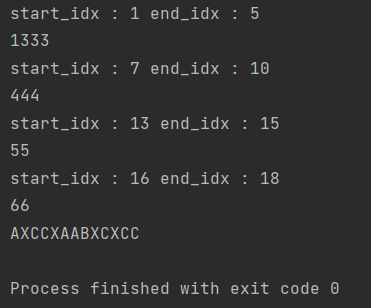
'Java > Basic' 카테고리의 다른 글
Java 람다식 (0) | 2023.04.05 |
---|---|
Java 익명 클래스 (0) | 2023.04.04 |
[JVM - 2] ClassLoader (0) | 2022.10.25 |
Java 정렬 (0) | 2022.09.07 |
[JVM - 1] JVM, JRE, JDK (0) | 2022.08.17 |